A Complete Guide to the ChatGPT API

Maxwell Timothy
Nov 18, 2024
13 min read
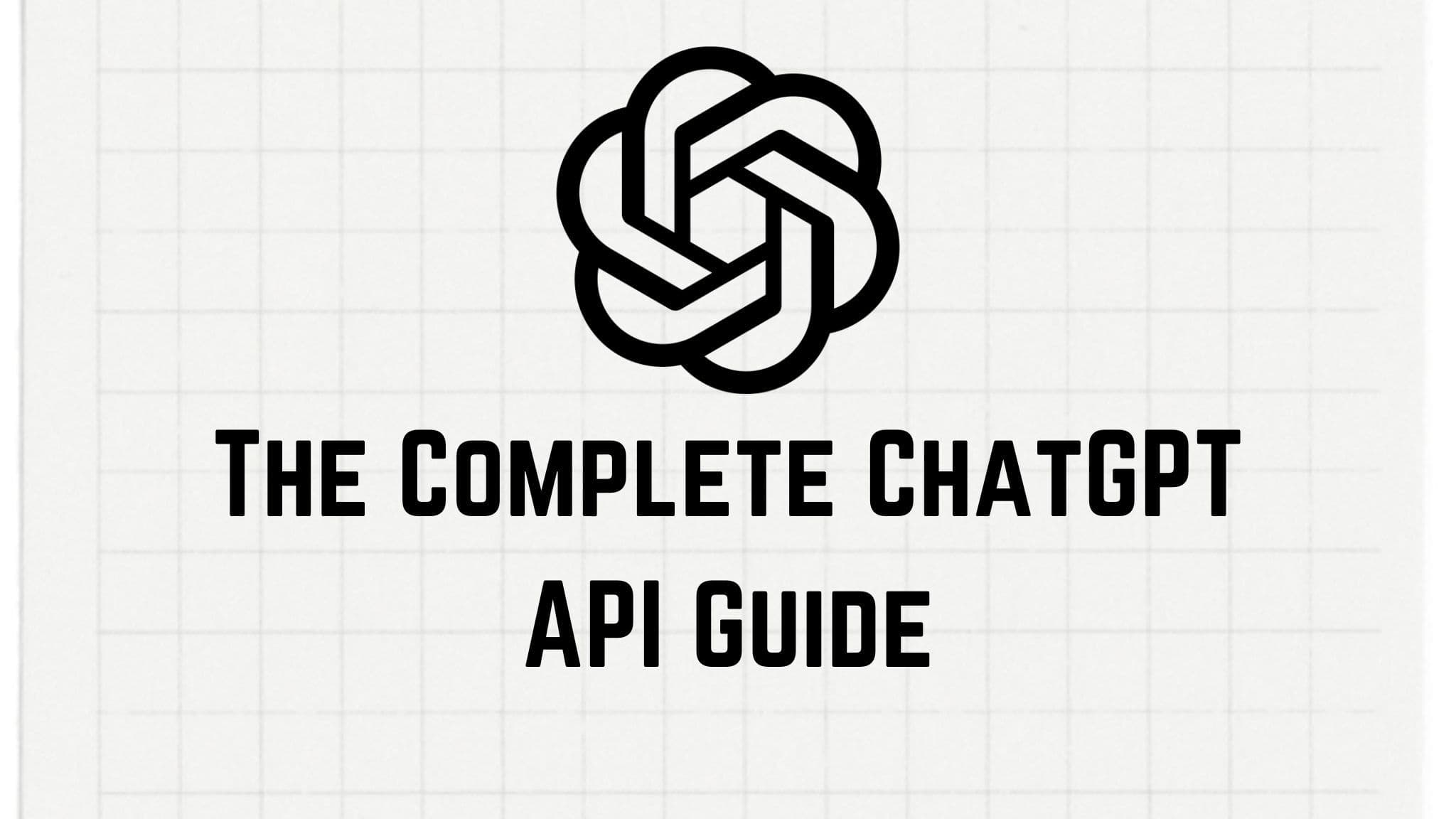
Are you looking to start building with the ChatGPT API?
This guide is designed for anyone who wants to explore the full potential of OpenAI’s ChatGPT API. Whether you’re a developer integrating AI into an existing application or creating something entirely new, we’ve got you covered.
You’ll learn how to obtain your API key, set up your development environment, and configure the ChatGPT API for different use cases.
By the end of this guide, you’ll have a clear understanding of how to use the ChatGPT API to build powerful, AI-driven applications.
What’s the ChatGPT API?
The ChatGPT API is a gateway tool that allows developers to integrate OpenAI’s powerful language models into their applications.
It provides access to GPT-based models like GPT-4, GPT-4-Turbo, GPT-4o, etc, enabling applications to understand and generate human-like text.
The API is optimized for a variety of tasks, such as chat-based interactions, text completions, language translation, code generation, etc. By using the ChatGPT API, you can add AI-driven features to your app, whether it’s creating chatbots, writing marketing copy, or developing tools that process natural language.
OpenAI has made the ChatGPT API flexible, allowing you to configure it for different use cases through parameters like model type, temperature, and response length.
With the right setup, you can customize the behavior and output of the API to fit your specific application needs.
How to Set Up the ChatGPT API
To start building with the ChatGPT API, you’ll need to set up your environment correctly. This involves getting access to OpenAI’s services, configuring your development tools, and testing your connection.
Whether you’re an experienced developer or just starting out, following these steps will help you smoothly set up the ChatGPT API and prepare for powerful integrations.
1. How to Obtain an OpenAI API Key
The API key is essential for accessing the ChatGPT API. Think of it as your personal identification card, granting you permission to interact with OpenAI’s models. Without it, you won’t be able to send requests or receive responses from the API. Here’s how to obtain it:
Step 1: Sign Up or Log In
- Visit the OpenAI platform.
- If you’re new, create an account by signing up. Returning users can log in.
Step 2: Navigate to the API Keys Section
- Once logged in, look for the API Keys tab in the left-hand menu of your OpenAI dashboard. This is where you can manage and create keys for your projects.
Step 3: Generate a New Key
- Click on the Create new secret key button.
- A new API key will be generated and displayed.
Step 4: Save Your API Key Securely
- Important: You can only view your key once when it’s generated. Copy it immediately and save it in a secure location, such as a password manager or a .env file in your development environment.
- If you lose it, you’ll need to generate a new key.
Why is the API Key Important?
The API key links your requests to your OpenAI account and ensures proper billing. It also defines your access permissions for various models, including GPT-3.5 Turbo, GPT-4, and other features.
By completing these steps, you’ve taken the first step toward setting up the ChatGPT API. Next, you’ll need to configure your development environment to start building.
Setting Up Your Development Environment
Once you have your OpenAI API key, the next step is to set up your development environment. This process ensures you’re ready to make API calls, write custom code, and integrate the ChatGPT API into your applications.
Developers can choose from several programming languages to work with the API, each with its own advantages. Let’s briefly explore some of the popular options:
1. JavaScript: Flexibility for Web Applications
JavaScript is one of the most widely used programming languages for building web applications. It integrates seamlessly with frameworks like Node.js and React, making it a great choice for developers building browser-based or real-time applications. Additionally, JavaScript’s asynchronous capabilities work well with API requests, ensuring smooth performance.
2. Python: Simplicity and a Rich Ecosystem
Python stands out for its simplicity and readability, making it ideal for beginners and experts alike. It boasts a vast library ecosystem, including packages like openai, which streamline working with the ChatGPT API. Python is also versatile, being used in everything from machine learning to web development.
3. Java: Scalability and Performance
Java is a robust and high-performance language, ideal for enterprise-level applications. It’s commonly used in environments where stability, scalability, and security are critical. Java’s multi-threading capabilities also allow for handling multiple API requests simultaneously, making it a strong option for large-scale systems.
For this demo, we’ll be using python to demonstrate how to build with the ChatGPT API.
Why Choose Python for ChatGPT API Development?
While all of these languages are powerful, Python is often the preferred choice for working with the ChatGPT API. Its simplicity makes it beginner-friendly, while its extensive library ecosystem, including the openai and python-dotenv packages, enables developers to get started quickly.
If you’re new to working with APIs or want to build a functional prototype fast, Python is the way to go.
Below, we’ll walk you through setting up your development environment using Python.
How to Set Up a Python Development Environment for ChatGPT API
You can follow the steps below to configure Python for working with the ChatGPT API:
Step 1: Install Python
- If Python isn’t already installed on your machine, download it from the official Python website.
- Ensure you’re using Python 3.7 or higher, as it’s required for many libraries.
Step 2: Create a Virtual Environment
A virtual environment isolates your project dependencies, ensuring that your API project doesn’t interfere with other Python projects.
To create a virtual environment:
python -m venv chatgpt-env
On Windows:
chatgpt-env\Scripts\activate
On macOS/Linux:
source chatgpt-env/bin/activate
Step 3: Install Required Libraries
Next, install the libraries needed to work with the ChatGPT API:
install
1pip install openai python-dotenv
The openai library allows you to interact with the API while the python-dotenv library helps securely manage your API key.
Step 4: Store Your API Key Securely
Create a .env file in your project’s root directory. This file will store your API key securely:
API key variable
1OPENAI_API_KEY="your-api-key-here"
To ensure your .env file isn’t shared accidentally, add it to your .gitignore file:
.env
Step 5: Test Your Setup
Write a simple script to confirm that your environment is working:
simple test script
1import openai2from dotenv import load_dotenv3import os45# Load API key from .env file6load_dotenv()7api_key = os.getenv("OPENAI_API_KEY")89# Initialize OpenAI client10openai.api_key = api_key1112# Make a simple API call13response = openai.ChatCompletion.create(14 model="gpt-4o",15 messages=[{"role": "user", "content": "Say hi to Chatbase!"}]16)1718# Print the response19print(response.choices[0].message.content)
Run the script. If everything goes well, you should see an API response like this:
API response
1ChatCompletion(2 id='chatcmpl-9yHksQWtvnXpK657az004k72YZLMNd',3 choices=[4 Choice(5 finish_reason='stop',6 index=0,7 logprobs=None,8 message=ChatCompletionMessage(9 content='{\n "joke": "Why do Java developers wear glasses? Because they don\'t C#!"\n}',10 role='assistant',11 function_call=None,12 tool_calls=None13 )14 )15 ],16 created=1707385354,17 model='gpt-4o',18 object='chat.completion',19 system_fingerprint='fp_2hgrts76b9',20 usage=CompletionUsage(21 completion_tokens=20,22 prompt_tokens=68,23 total_tokens=8824 )25)26
You can use the code below to extract the relevant response from the API response:
print response
1print(response.choices[0].message.content)
And, in this case, you should have something like:
response extract
1{"joke": "Why do Java developers wear glasses? Because they don't C#!}'
ChatGPT API Advanced Tips and Tricks
Now that you’ve set up your development environment, it’s time to level up your use of the ChatGPT API. These advanced tips and tricks will help you optimize performance, save costs, and create more effective interactions. Let’s dive in.
1. Use Temperature and Max Tokens Wisely
The temperature and max_tokens parameters control your API’s behavior.
- Temperature: Adjust the creativity of the responses.
- Lower values (e.g., 0.2) make responses more focused and deterministic—ideal for factual answers or structured outputs.
- Higher values (e.g., 0.8) introduce more randomness, which works well for creative applications.
- Max Tokens: Limit the response length.
- Reducing max_tokens saves costs and ensures concise outputs.
- Always calculate the token budget for both input and output to avoid truncation.
Start with defaults, then tweak based on your application needs. Experiment to find the right balance.
2. Structure Prompts Effectively
The way you structure your prompt heavily influences the quality of responses.
- Be specific: Include detailed instructions about the task. For example:
- Instead of: “Explain photosynthesis.”
- Use: “Explain photosynthesis in simple terms for a 5th-grade student, using examples.”
- Use examples: Provide context to guide the API’s output. Example:
- “Rephrase this sentence in formal language: ‘Can you fix this issue?’ Output: ‘Would you mind resolving this issue?’”
- Role-based prompts: Add context by assigning a role to ChatGPT. For example:
- “You are a professional copywriter. Write a compelling product description for a smartwatch.”
Clear, structured prompts lead to precise, relevant answers.
3. Handle Long Conversations with Token Management
ChatGPT has a token limit, so it’s critical to manage long conversations efficiently.
- Trim unnecessary tokens: Only keep essential messages in the messages array.
- Use summarization: Regularly summarize the conversation and replace older messages with the summary.
- Monitor token usage: Use the tiktoken library to count tokens in advance and avoid errors caused by exceeding the token limit.
This will help you get a smooth interaction, even in long-running conversations.
4. Implement Rate Limiting
If your application sends frequent API requests, you may run into rate limits. Avoid this by:
- Batching requests: Combine smaller requests into a single, larger one when possible.
- Using retry logic: Automatically retry failed requests after a short delay.
- Scaling up your plan: Upgrade your OpenAI plan if you frequently hit rate limits.
5. Optimize Costs
Working with the ChatGPT API can get expensive if not managed carefully. Here’s how to minimize costs:
- Choose the right model: Use gpt-4o mini instead of gpt-4-Turbo for less complex tasks. It’s faster and significantly cheaper.
- Limit output size: Use shorter prompts and max_tokens to reduce token consumption.
- Cache results: Store API responses for frequently asked questions or static outputs instead of querying repeatedly.
I strongly recommend you check the OpenAI API pricing page to know the cost of each model before you start building any production application with it.
Regularly monitor your API usage to identify areas where you can cut costs.
6. Use Function Calling for Structured Data
If your application needs structured responses, leverage the function calling feature.
- Define your function’s schema and pass it to the API. For example:
- “Extract the user’s name, email, and message from this text and return it as a JSON object.”
Function calling ensures the API outputs data in a predictable and easily parsable format, saving time and effort during post-processing.
7. Implement Fail-Safe Systems
API calls can occasionally fail. Be prepared for this by:
- Using timeout handling: Retry the request if it times out.
- Creating fallback responses: Show a user-friendly error message if the API fails.
- Adding logging: Track failures to debug and improve reliability.
Fail-safes maintain a seamless experience for your users, even when things go wrong.
8. Experiment with Fine-Tuning
For highly specific use cases, consider fine-tuning the model. This allows you to train the API on your own data for customized responses.
- Prepare a dataset with clear input-output pairs.
- Use OpenAI’s fine-tuning documentation to upload and train your model.
- Test the fine-tuned model thoroughly before deploying it.
Fine-tuning can drastically improve performance for niche applications but should only be used when necessary.
9. Secure Your API Key
Protect your OpenAI API key to avoid unauthorized use.
- Store it in a .env file or environment variables, never in your codebase.
- Rotate your key periodically for added security.
- Use OpenAI’s API usage dashboard to monitor and detect unusual activity.
Security is critical to prevent misuse and unexpected costs.
App Ideas to Build with ChatGPT API
Once you’ve mastered the ChatGPT API, there are a lot of very interesting and powerful apps you can build. From personal productivity tools to complex enterprise solutions, the ChatGPT API allows you to build applications that fit your needs. Let’s look at some of the most common types of apps you can create.
1. Chatbots for Customer Support
Customer support is one of the most popular applications of the ChatGPT API. You can create chatbots that:
- Answer FAQs quickly and accurately.
- Troubleshoot common issues.
- Help customers track orders, manage bookings, or process refunds.
With features like context handling and function calling, your chatbot can go beyond basic responses by integrating with external systems like ticketing platforms or CRMs.
2. Virtual Assistants for Productivity
The ChatGPT API can power virtual assistants that streamline everyday tasks. These assistants can:
- Schedule meetings by syncing with Google Calendar.
- Set reminders and alarms.
- Summarize documents, articles, or emails.
3. AI-Powered Educational Tools
Educational apps powered by the ChatGPT API can offer personalized learning experiences. Examples include:
- Language learning apps that simulate real-world conversations.
- Study tools that explain complex topics in simple terms.
- Interactive tutors that answer student questions and provide feedback on essays or coding exercises.
One of the things AI will bring to the table in apps like these is that it is going to make it better tailored for different age groups, skill levels, or subjects, making learning more engaging.
4. Content Creation Tools
Content creation is another powerful use case for the ChatGPT API. Developers can build tools to:
- Write blog posts, social media captions, or marketing copy.
- Generate scripts for videos or presentations.
- Assist with creative writing, like brainstorming story ideas or crafting creative content.
5. Personalized Recommendation Systems
Use the ChatGPT API to create systems that offer personalized recommendations. For example:
- E-commerce apps that suggest products based on user preferences.
- Entertainment apps that recommend movies, books, or music.
- Lifestyle apps that provide travel itineraries or fitness routines.
6. Data Analysis Tools
Develop apps that help users analyze and understand data. The ChatGPT API can:
- Simplify complex datasets by summarizing trends and insights.
- Answer natural language queries.
These tools are especially useful for businesses looking to make data-driven decisions without needing advanced technical skills.
7. Code Generation and Debugging Tools
For developers, the ChatGPT API can help with building coding tools. Use it to build apps that:
- Write code snippets in multiple programming languages.
- Debug code by identifying errors and suggesting fixes.
- Generate explanations for how a particular block of code works.
8. Games and Interactive Story Apps
The ChatGPT API can bring interactive entertainment to life. Examples include:
- Text-based adventure games where the player interacts with the story dynamically.
- AI dungeon masters for tabletop RPGs.
- Interactive fiction apps where users co-create a story with AI.
9. Knowledge Bases and Search Tools
Build apps that improve access to information. Examples include:
- Internal company knowledge bases like Document360 with natural language search.
- Research tools that summarize academic papers or documents.
- Industry-specific assistants for legal, medical, or financial queries.
When paired with relevant datasets using techniques like RAG, these apps offer highly specialized and reliable insights.
With the ChatGPT API, your app’s functionality is limited only by your imagination. My advice? Start small, experiment, and scale as your application grows.
Ready to Build?
The ChatGPT API is a powerful tool for building intelligent applications, from chatbots to productivity tools and interactive experiences. Its advanced natural language capabilities empower developers to create apps that are contextual, intuitive, and scalable.
But building truly powerful apps often requires more than just raw API access. If you want to go beyond generic AI responses and integrate your app with custom data, things can quickly get complicated.
The Chatbase API simplifies this process by leveraging Retrieval-Augmented Generation (RAG) to connect your chatbot or app to your data, ensuring responses are accurate and contextually relevant. It eliminates the complexities of handling custom data, enabling you to focus on building powerful applications, like chatbots for e-commerce or customer support, without worrying about backend pipelines.
With Chatbase, you combine the flexibility of the ChatGPT API with the ease of building apps grounded in your own data. Sign up for Chatbase today and start creating smarter, faster, and more customized AI-powered apps.
Share this article: