How to Access and Use the DeepSeek API

Maxwell Timothy
Apr 11, 2025
10 min read
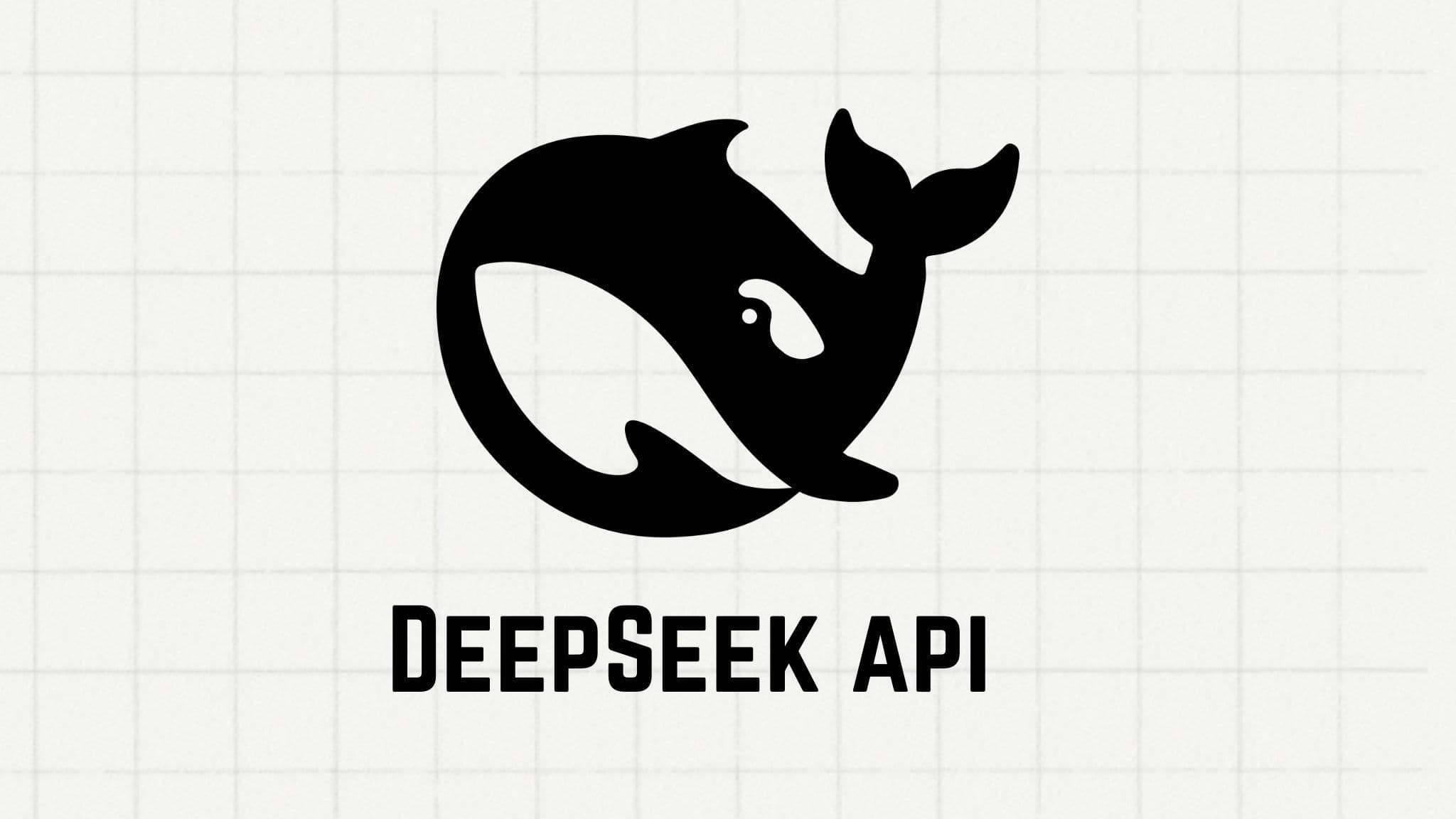
There’s been a lot of buzz around DeepSeek lately—and for good reason. It’s one of those open-source projects that showed up and instantly got people talking. Some folks were skeptical at first (as always), but many quickly realized DeepSeek isn’t just another flash-in-the-pan release.
It’s fast, surprisingly capable, and positioned itself as a serious alternative to the big-name models. And when you consider that it's fully open-source, things start getting even more interesting.
But hype aside, let’s talk practicality.
If you’re building something—whether it’s a personal tool, a production-grade product, or just running a few experiments—and you’re curious how DeepSeek fits in, this guide will walk you through it all.
We'll cover the models, how to get set up, and how to actually use DeepSeek in your project. Nothing fancy, no fluff—just what you need to get started, quickly and clearly.
The DeepSeek Models
When people talk about DeepSeek, they’re usually referring to one of two main models: DeepSeek-V3, also called DeepSeek Chat, and DeepSeek-R1, known as DeepSeek Reasoner. These are the two models we’ll be working with.
They each serve a different purpose, so understanding what makes them different is important if you're trying to choose the right one for your use case.
DeepSeek-V3 (DeepSeek Chat)
This is DeepSeek’s general-purpose model.
It was trained on a massive dataset—over 15 trillion tokens. That’s a lot. For context, most mainstream large language models are trained on fewer tokens. The idea here is to expose the model to as much text as possible across a wide range of domains.
That’s why V3 works well across a broad set of tasks—writing, chatting, summarizing, answering questions, even some light coding and reasoning.
If you're building a chatbot, a writing assistant, or anything that needs to understand and respond naturally to prompts, this is your go-to.
DeepSeek-R1 (DeepSeek Reasoner)
R1 is a different beast.
It’s built for more specialized reasoning tasks like advanced math, logic, coding, and multi-step problem-solving.
This model hasn’t just seen a lot of data, it’s also been fine-tuned to perform deeper reasoning. You’d want to use this one for more technical agents, internal tools that need accuracy, or tasks where step-by-step thinking really matters.
How to Access the DeepSeek API
If you're looking to plug DeepSeek into your own projects, here's how to do it without getting lost in setup docs. From grabbing your API key to making your first call, this guide breaks it all down clearly.
Step 1: Get Your API Key
Go to the DeepSeek API Platform and sign up.
Once you're in, head over to the API Key section. There, you can create and manage your keys.
Important heads-up: your key is only shown once at the moment of creation. Copy it and keep it somewhere safe—you won’t be able to see it again. And yeah, don’t share it publicly unless you’re looking for headaches.
Step 2: Use the OpenAI SDK (Yes, Really)
DeepSeek is fully compatible with the OpenAI SDK, so you won’t need to reinvent the wheel.
The OpenAI SDK is basically a toolkit that gives you pre-built functions to make API calls easier. You don’t have to worry about raw HTTP requests or complex configs—it handles all of that under the hood.
Step 3: Make Your First API Call
Here’s the basic code to get you connected:
api call
1from openai import OpenAI2client = OpenAI(3api_key="<DeepSeek API Key>",4base_url="https://api.deepseek.com"5)6response = client.chat.completions.create(7model="deepseek-chat",8messages=[9{"role": "system", "content": "You are a helpful assistant"},10{"role": "user", "content": "Hello"},11],12stream=False13)14print(response.choices[0].message.content)
A few things to point out here:
- Replace <DeepSeek API Key> with your actual key.
- Set model="deepseek-chat" to use DeepSeek V3.
- Use model="deepseek-reasoner" if you want to switch over to DeepSeek R1 instead.
Quick Code Breakdown
- from openai import OpenAI: Brings in the OpenAI SDK.
- client = OpenAI(...): Sets up your connection using your API key and DeepSeek's base URL.
- messages=[...]: This is the chat history. You set the system behavior and the user input.
- stream=False: Tells the model to return the full response in one go (you can set it to True if you want a live-streaming effect).
- print(...): Pulls the model's reply from the response object and prints it.
Prompt Tweaks That Cut Token Use
If you’re trying to get the most out of DeepSeek’s models without racking up high token bills, there are a few levers you can pull: temperature, formatting, and Chain-of-Thought reasoning. Here’s how each one works — and how to use them to your advantage.
Adjusting Temperature Based on Use Case
Temperature controls how creative or predictable the model's responses are. DeepSeek defaults to 1.0, which works well for general use. But certain tasks benefit from tuning this number.
For example, when generating code or solving math problems, a temperature of 0.0 keeps things focused and consistent. For data-related tasks like cleaning or transformation, staying at 1.0 offers a balance between precision and flexibility. If you're handling translation or general back-and-forth conversations, bumping it up to 1.3 helps the model loosen up a bit. And for fiction writing or creative ideation, setting temperature to 1.5 unlocks the full expressive range.
Lower temperatures reduce randomness, making answers more direct — but less diverse. Higher temperatures invite more variety, at the cost of potential rambling. Always keep in mind: longer, more creative outputs = more tokens = higher cost.
Formatting Responses to Stay Lean
You can shape the output by specifying response_format={'type': 'structured_json'} in your API call. This tells DeepSeek to return answers in clean JSON instead of long paragraphs.
Structured formatting has two key benefits: it keeps replies short and scannable (which lowers token usage), and it makes integration with your application far easier. It’s not just about clean code — it’s also about not paying for unnecessary fluff in free-form text. When you’re running lots of queries, those savings add up.
Chain-of-Thought: Power Comes at a Cost
Chain-of-Thought (CoT) reasoning is what makes deepseek-reasoner special. It allows the model to "think through" a problem before answering. This improves accuracy for complex or multi-step questions — but the catch is, it outputs more text.
Since every step in that reasoning chain costs tokens (billed at $2.19 per million), you need to use CoT wisely. Avoid prompting for long-winded breakdowns. Instead, ask for brief logic or a summary of reasoning. If you're solving simple tasks, stick with deepseek-chat to keep things snappy and affordable.
Also, don’t forget to enable caching — especially when repeating similar prompts — to keep input costs under control.
Example: Triggering CoT with a Prompt
Here’s a sample setup for deepseek-reasoner where the model is encouraged to walk through its logic:
api call
1from openai import OpenAI2client = OpenAI(3api_key="<Your_DeepSeek_API_Key>",4base_url="https://api.deepseek.com"5)6def fetch_reasoned_reply(query):7response = client.chat.completions.create(8model="deepseek-reasoner",9messages=[10{"role": "system", "content": "Act as a logic-driven assistant."},11{"role": "user", "content": query}12],13stream=False14)15return response.choices[0].message.content16# Test example17query = "What is the total surface area of a cube with a 5 cm edge length?"18output = fetch_reasoned_reply(query)19print(output)
With a query like this, you’ll usually get a step-by-step breakdown — e.g., calculating 6 × edge² — before the final result. That makes it perfect for tasks where accuracy matters, even if it costs a bit more.
Making Chain-of-Thought Work Without Wasting Tokens
Chain-of-Thought (CoT) prompts are powerful for reasoning tasks, but if you’re not careful, they’ll burn through your token budget fast. Let’s look at how a small tweak can make a big cost difference.
Overexplaining Can Get Pricey
Here’s what happens when you let the model think freely without constraints:
Prompt: “How do I find the greatest common divisor (GCD) of 48 and 18?”
Model Output: To find the GCD, I can use the Euclidean algorithm. First, divide 48 by 18, which gives 2 with a remainder of 12. Then divide 18 by 12, which gives 1 with a remainder of 6. Then divide 12 by 6, which gives 2 with a remainder of 0. The GCD is the last non-zero remainder, which is 6. Let me check again: 48 % 18 = 12, 18 % 12 = 6, 12 % 6 = 0. Yep, it’s 6. Much faster than listing divisors manually.
Tokens used: Around 910 Cost estimate: ~$0.00199 What went wrong: The model is narrating every detail, adding redundancy, and even doing a double check.
A Small Prompt Fix = Big Savings
You don’t need all that explanation if your goal is just the result. Here’s a cleaner version of the same task:
Prompt: “Use the Euclidean algorithm to find the GCD of 48 and 18. Return steps and result only.”
Model Output: 48 % 18 = 12 18 % 12 = 6 12 % 6 = 0 GCD = 6
Tokens used: Around 150 Cost estimate: ~$0.00033 What worked: Clear instruction to keep it short and structured.
How to Keep CoT Efficient
Tell the model exactly what format you want. Phrases like “don’t explain,” “show steps only,” or “final answer only” go a long way. Use method-based instructions (e.g., “solve using Euclidean algorithm”) to guide the reasoning. The more vague your prompt, the more verbose and costly the model gets.
Less Chatter, Same Value
If you’re running CoT prompts at scale, this kind of prompt discipline can save you real money—without sacrificing accuracy. Tighter prompts = fewer tokens = lower cost. Simple as that.
Skip the Hassle—Use DeepSeek with Chatbase Instead
You don’t need to wrestle with APIs, fine-tuning scripts, or infrastructure just to get DeepSeek working with your own data. If you’re building a chatbot, a conversational AI tool, or even an internal agent powered by DeepSeek, Chatbase makes the entire process effortless.
Just sign up on Chatbase, create a new chatbot or agent, and upload your files or documents. That’s it. Chatbase will automatically train your AI on that knowledge and power it using DeepSeek under the hood.
Whether you're looking to connect it to your app, integrate it into your website, or access it via API, you can get everything running in 5–10 minutes. No complicated setup. No code-heavy workflow. Just a powerful DeepSeek chatbot, trained on your data and ready to deploy.
→ Sign up for Chatbase now and start building your DeepSeek-powered AI agent in minutes.
Share this article: