Document Parsing: Techniques, Tools, and Best Practices

Ilias Ism
Sep 29, 2024
12 min read
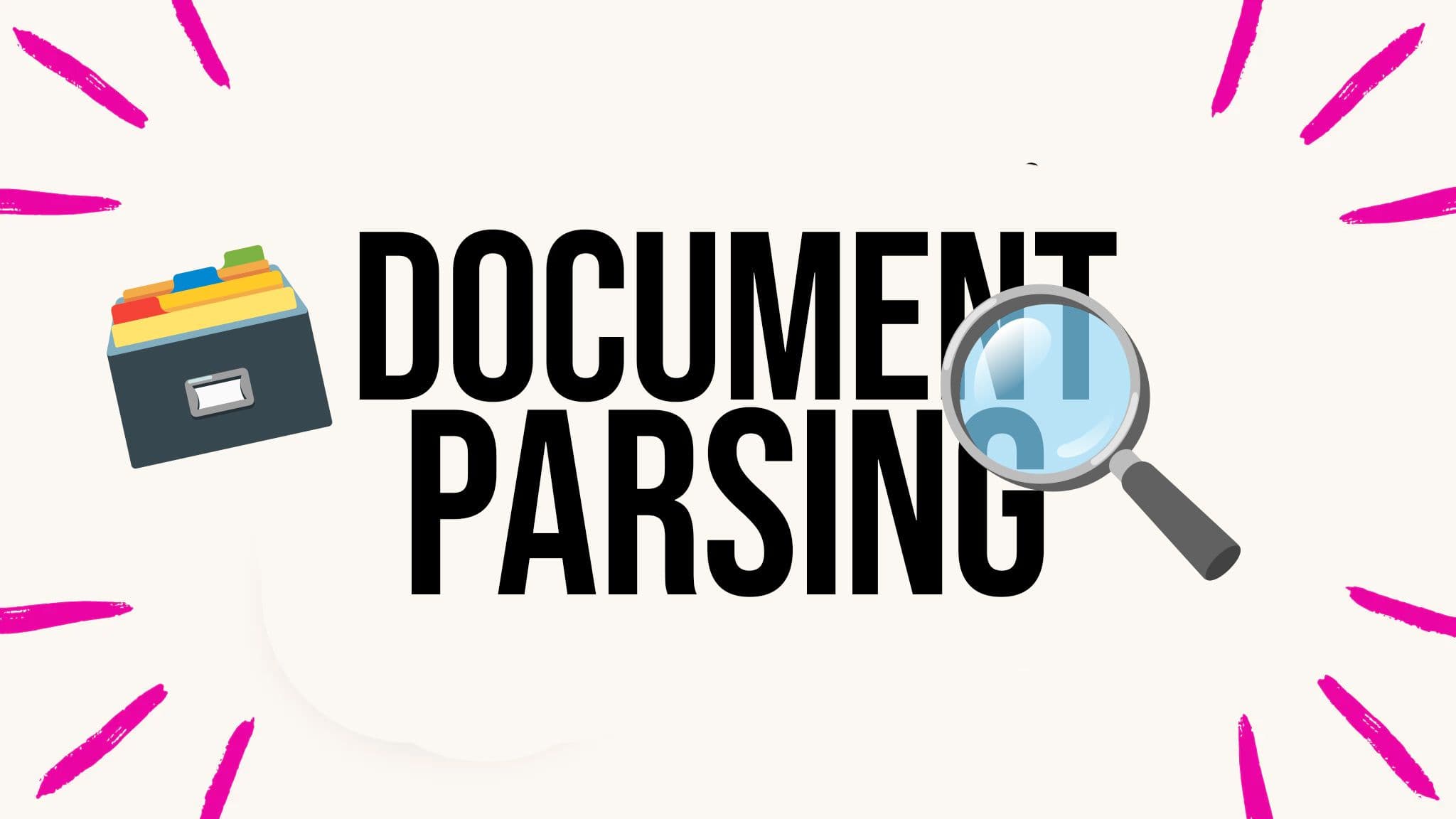
4Document parsing is a crucial process in the modern data-driven world, enabling organizations to extract valuable information from unstructured or semi-structured documents.
This comprehensive guide will explore various document parsing techniques, tools, and best practices to help you efficiently extract and process data from diverse document types.
What is Document Parsing?
Document parsing is the process of extracting structured data from unstructured or semi-structured documents.
It involves analyzing the content and structure of a document to identify and extract specific pieces of information.
This process is essential for automating data entry, improving data accuracy, and enabling efficient information retrieval.
Key Techniques for Document Parsing
1. Regular Expressions (Regex)
Regular expressions are powerful tools for pattern matching and text extraction.
They allow you to define specific patterns to search for within a document and extract matching content.
Here's a Python example using regex to extract email addresses from a text document:
extract.py
1import re23def extract_emails(text):4 pattern = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,7}\b'5 return re.findall(pattern, text)67sample_text = "Contact us at john.doe@example.com or support@company.org for assistance."8emails = extract_emails(sample_text)9print(emails)10# Output: ['john.doe@example.com', 'support@company.org']
This regex pattern matches the structure of email addresses, allowing you to quickly extract them from any text document.
2. Natural Language Processing (NLP) with spaCy
spaCy is a powerful NLP library that can be used for various document parsing tasks, including named entity recognition, part-of-speech tagging, and dependency parsing.
Here's an example of using spaCy to extract named entities from a text document:
spacy.py
1import spacy23nlp = spacy.load("en_core_web_sm")45def extract_entities(text):6 doc = nlp(text)7 entities = [(ent.text, ent.label_) for ent in doc.ents]8 return entities910sample_text = "Apple Inc. was founded by Steve Jobs in Cupertino, California in 1976."11entities = extract_entities(sample_text)12print(entities)13# Output: [('Apple Inc.', 'ORG'), ('Steve Jobs', 'PERSON'), ('Cupertino', 'GPE'), ('California', 'GPE'), ('1976', 'DATE')]
This example demonstrates how spaCy can automatically identify and classify named entities in the text, which is particularly useful for extracting specific types of information from documents.
3. Machine Learning-based Approaches
Machine learning models, particularly deep learning models, have shown great promise in document parsing tasks.
These models can be trained on large datasets to recognize patterns and extract information from various document types.
One popular approach is to use Convolutional Neural Networks (CNNs) for document layout analysis and Recurrent Neural Networks (RNNs) or Transformers for text extraction and classification.
4. Rule-based Systems
Rule-based systems involve creating a set of predefined rules to identify and extract specific information from documents.
While less flexible than machine learning approaches, rule-based systems can be highly effective for documents with consistent structures.
Advanced Document Parsing with AI-powered Tools
1. ChatGPT for Unstructured Text Parsing
ChatGPT, powered by OpenAI's language models, can be an incredibly powerful tool for parsing unstructured text.
Its natural language understanding capabilities allow it to extract relevant information from complex documents with high accuracy.
Here's an example of using ChatGPT to parse unstructured text:
chatgpt.py
1import openai23openai.api_key = 'your_api_key_here'45def parse_text_with_chatgpt(text):6 prompt = f"Extract the following information from the text below:\n\n{text}\n\nProvide the output as JSON with the following fields: name, email, phone_number, company, position"78 response = openai.Completion.create(9 engine="text-davinci-002",10 prompt=prompt,11 max_tokens=15012 )1314 return response.choices.text.strip()1516sample_text = """17John Smith18Senior Software Engineer19TechCorp Inc.20Email: john.smith@techcorp.com21Phone: (555) 123-456722"""2324parsed_data = parse_text_with_chatgpt(sample_text)25print(parsed_data)
This example demonstrates how ChatGPT can be used to extract structured information from unstructured text, providing a flexible and powerful solution for document parsing tasks.
2. Claude 3.5 with Zod for Structured Data Validation
Claude 3.5, developed by Anthropic, is another powerful AI model that can be used for document parsing.
When combined with Zod, a TypeScript-first schema declaration and validation library, it provides a robust solution for extracting and validating structured data from documents.
Here's an example of using Claude 3.5 with Zod for parsing and validating structured data:
claude.ts
1import { z } from 'zod';2import { Claude } from 'anthropic';34const claude = new Claude({ apiKey: 'your_api_key_here' });56const PersonSchema = z.object({7 name: z.string(),8 email: z.string().email(),9 phone: z.string(),10 company: z.string(),11 position: z.string(),12});1314async function parseAndValidateWithClaude(text: string) {15 const prompt = `Extract the following information from the text below and format it as JSON:\n\n${text}\n\nProvide the output with the following fields: name, email, phone, company, position`;1617 const response = await claude.complete({18 prompt,19 max_tokens_to_sample: 200,20 });2122 const parsedData = JSON.parse(response.completion);23 return PersonSchema.parse(parsedData);24}2526const sampleText = `27John Smith28Senior Software Engineer29TechCorp Inc.30Email: john.smith@techcorp.com31Phone: (555) 123-456732`;3334parseAndValidateWithClaude(sampleText)35 .then((result) => console.log(result))36 .catch((error) => console.error(error));
This example showcases how Claude 3.5 can be used to extract structured data from text, while Zod ensures that the extracted data conforms to the expected schema.
Best Practices for Document Parsing
- Preprocessing: Clean and normalize your documents before parsing to improve accuracy. This may include removing noise, standardizing formats like converting heic to jpg, and handling encoding issues.
- Modular Design: Design your parsing system in a modular fashion, allowing for easy updates and maintenance of individual components.
- Error Handling: Implement robust error handling mechanisms to deal with unexpected document formats or content.
- Scalability: Design your parsing system to handle large volumes of documents efficiently, considering parallelization and distributed processing when necessary.
- Continuous Improvement: Regularly update and refine your parsing models or rules based on new data and feedback to improve accuracy over time.
- Privacy and Security: Ensure that your document parsing system adheres to data privacy regulations and implements appropriate security measures to protect sensitive information.
- Validation: Implement thorough validation checks on the extracted data to ensure accuracy and consistency.
- Human-in-the-Loop: For critical applications, consider implementing a human review process for parsed data to catch and correct any errors.
Conclusion
Document parsing is a critical process in today's data-driven world, enabling organizations to extract valuable insights from unstructured and semi-structured documents.
By leveraging techniques such as regex, NLP, machine learning, and AI-powered tools like ChatGPT and Claude 3.5, you can build powerful document parsing systems that efficiently extract and process information from diverse document types.
Remember to follow best practices, such as preprocessing your documents, implementing modular designs, and continuously improving your parsing models.
With the right approach and tools, you can transform your document parsing workflows, improving efficiency, accuracy, and data-driven decision-making across your organization.
As you embark on your document parsing journey, keep exploring new techniques and tools to stay at the forefront of this rapidly evolving field.
The future of document parsing holds exciting possibilities, with advancements in AI and machine learning promising even more accurate and efficient solutions for extracting valuable information from the ever-growing sea of digital documents.
Share this article: